Get To Know Babel The JavaScript Compiler Tutorial
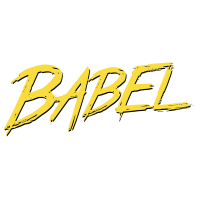
An updated Tutorial using Babel 6 can be found here!
Babel is a JavaScript transpiler. What that means is that we can take ES2015 code and make it work on all browsers, even ones that may not support it. Essentially Babel takes the ES2015 JavaScript code and compiles it into ES5 code.
This opens up all the ECMAScript 6 features to us when we code our projects. This includes features like arrows, classes, template strings, enhanced object literals, desctructuring and more. Check out my top six ES6 post I did earlier this year to get an idea on how all these new features. I also wrote something similar when I discussed TypeScript. You can check out my tutorial on that here.
For this tutorial we'll be using the Babel CLI. This is a command line tool that can be used to take existing JavaScript code and convert it from ES2015 to ES5 on the command line. This is just one of many tools you can use if you want to use Babel. If you're using Gulp or Grunt it's really easy to add Babel as a task to be run during your build process. We'll discuss that briefly below.
Setup
To get started let's install Babel on the command line. We'll assume that you already have node or iojs installed. If not check out my getting started with iojs guide.
$ npm install -g babel
Done and done!
Quick Example With Classes
Let's create a ES2015 class file and see if we can use Babel to convert it.
// Polygon.js
class Polygon {
constructor(height, width) {
this.height = height;
this.width = width;
}
get area() {
return this.calcArea()
}
calcArea() {
return this.height * this.width;
}
}
We can now use the Babel CLI command and convert it. We can do this several ways
$ babel Polygon.js
If we run the command above we'll see on the screen the output of the conversion. Of course that doesn't help a lot so let's redirect it to a file.
$ babel Polygon.js > output.js
That's fine but there is also a number of arguments babel CLI uses. One of them is to create a file with the output.
$ babel Polygon.js -o output.js
This will do the same thing as the redirect. What if we wanted it to compile the code on the fly, as it changes? We can do that with the watch command.
$ babel Polygon.js --watch -o poly.js
Any changes that occur to Polygon.js while this command is running will be instantly reflected in the poly.js file.
If needed you can have an entire folder compiled and outputted to another folder.
$ babel src --out-dir lib
This will take all the JavaScript files in src and compile them to the lib folder.
To look at all the command that's offered run this command
$ babel -h
Gulp Task Example
You can learn more about this process at the Babel website but here is an example of using Babel in your build system using gulp. This assumes you already have gulp installed.
$ npm install --save-dev gulp-babel
Then the actual gulp file will look like this.
// gulpfile.js
var gulp = require("gulp");
var babel = require("gulp-babel");
gulp.task("default", function () {
return gulp.src("src/app.js")
.pipe(babel())
.pipe(gulp.dest("dist"));
});
The default task is executed whenever you run the gulp command. This default task will take the app.js file and compile it using Babel and then output it to the dist folder.
Grunt Example
Much like gulp the process to compile is very similar. First we have to install grunt-babel. This assumes you already have grunt installed.
$ npm install --save-dev grunt-babel
Now here is the grunt file.
require("load-grunt-tasks")(grunt); // npm install --save-dev load-grunt-tasks
grunt.initConfig({
"babel": {
options: {
sourceMap: true
},
dist: {
files: {
"dist/app.js": "src/app.js"
}
}
}
});
grunt.registerTask("default", ["babel"]);
As you can see above it's very similar to gulp. You just choose the src and destination and it does the compilation. I haven't used this too much so I would read up on grunt if you're interested in learning more.
What's Next?
I hope this gives you a brief introduction on Babel. I want to explore a lot more build tools in the future, so expect more articles on that! Take care!
Leave a comment below with any questions!